Scatter and Gather is one of the common Enterprise Integration Patterns (EIP). As one of the leading open-source integration platforms, the WSO2 Enterprise Integrator (EI) provides an out-of-the-box approach to achieve it using synapse mediators.
In this article, we will be checking two approaches to achieve the Scatter and Gather pattern using Iterate and Clone mediators respectively.
1.Using Iterate-Aggregate mediators
Iterate mediator splits the parent message into smaller messages and sends each child message to the backend service. Then the aggregate mediator can be used to combine responses from each backend call to a single message before responding to the client.
Here is a sample API service that uses the Iterate and Aggregate mediator combination.
<?xml version="1.0" encoding="UTF-8"?>
<api xmlns="http://ws.apache.org/ns/synapse" name="IterateAPI" context="/employees">
<resource methods="POST">
<inSequence>
<iterate id="employee_iterator" preservePayload="true" attachPath="json-eval($.employees)" expression="json-eval($.employees)">
<target>
<sequence>
<send>
<endpoint>
<http method="POST" uri-template="http://run.mocky.io/v3/a9d63220-8fa8-4021-add0-28a635e965b2" />
</endpoint>
</send>
</sequence>
</target>
</iterate>
</inSequence>
<outSequence>
<property name="info" scope="default">
<Aggregated_Response />
</property>
<aggregate id="employee_iterator">
<completeCondition>
<messageCount min="-1" max="-1" />
</completeCondition>
<onComplete expression="json-eval($)" enclosingElementProperty="info">
<respond />
</onComplete>
</aggregate>
</outSequence>
<faultSequence />
</resource>
</api>
The API service is designed to accept a JSON message and split the message into employee JSON objects, before sending it to the desired backend service.
Use the below payload to invoke the above API service.
{
"employees":[
{"emp_id":15693, "name":"Adrian"},
{"emp_id":16180, "name":"Andrea"},
{"emp_id":15025, "name":"Barry"}
]
}
You will get a response as below.
{
"Aggregated_Response": [
{"Response": "Success" },
{ "Response": "Success" },
{"Response": "Success" }
]
}
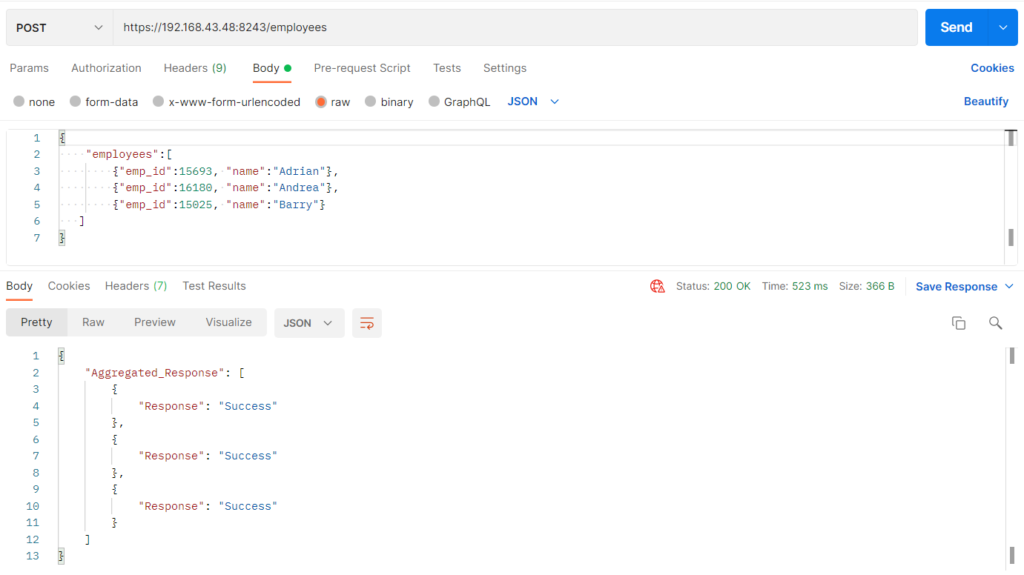
2. Using Clone-Aggregate mediators
Similar to the Iterate mediator, the clone mediator also can be used to send messages to multiple backends. The difference is that when the Iterate mediator splits the original message into child messages the clone mediator clones the original message and sends identical copies to the backend.
Try the below API service to understand the clone mediator functionality.
<?xml version="1.0" encoding="UTF-8"?>
<api xmlns="http://ws.apache.org/ns/synapse" name="CloneAPI" context="/employees">
<resource methods="POST">
<inSequence>
<clone>
<target>
<endpoint name="endpoint_1">
<http method="POST" uri-template="http://run.mocky.io/v3/6e367e76-e197-4ab3-a4c9-ea659b8ffbe9" />
</endpoint>
</target>
<target>
<endpoint name="endpoint_2">
<http method="POST" uri-template="http://run.mocky.io/v3/332f7d23-e4d3-427c-91bf-b8fd2d35c6df" />
</endpoint>
</target>
<target>
<endpoint name="endpoint_3">
<http method="POST" uri-template="http://run.mocky.io/v3/537616ea-355c-47f1-ad3c-64fa3378661f" />
</endpoint>
</target>
</clone>
</inSequence>
<outSequence>
<property name="info" scope="default">
<Aggregated_Response />
</property>
<aggregate>
<completeCondition>
<messageCount min="-1" max="-1" />
</completeCondition>
<onComplete expression="json-eval($)" enclosingElementProperty="info">
<respond />
</onComplete>
</aggregate>
</outSequence>
<faultSequence />
</resource>
</api>
Use the below payload to invoke the above API service.
{
"employees":[
{"emp_id":15693, "name":"Adrian"},
{"emp_id":16180, "name":"Andrea"},
{"emp_id":15025, "name":"Barry"}
]
}
You will get the below response.
{
"Aggregated_Response": [
{"Response": "From Backend 1"},
{"Response": "From Backend 2"},
{"Response": "From Backend 3"}
]
}
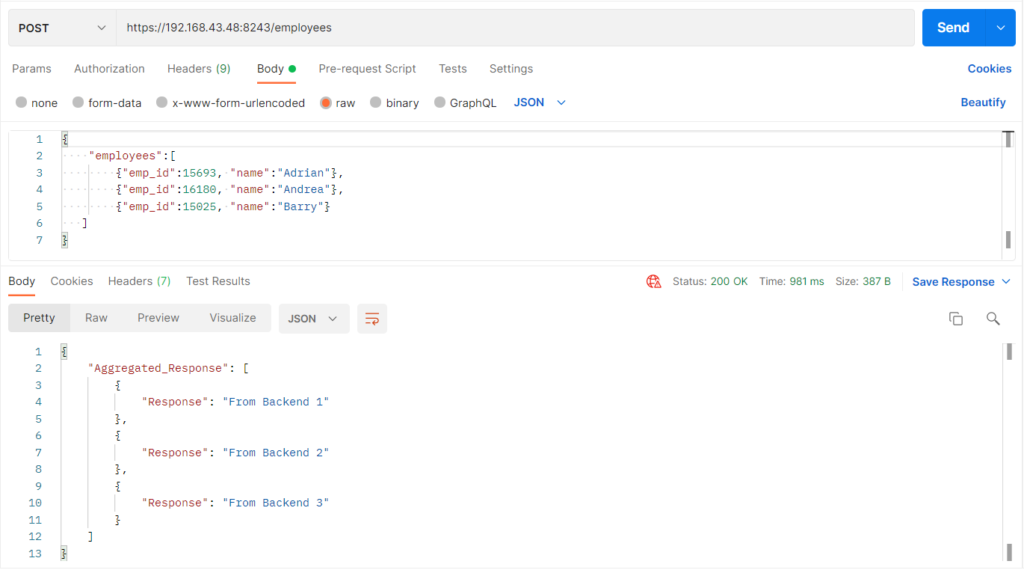
The Iterate mediator sends every request to the same backend service. But when using the Clone mediator, we can invoke different backend services parallelly and aggregate the response from each.
That’s it then.
Each approach has its pros and cons. Give both a try and see what suits you the most.
We suppose that you learned something out of this. Keep in touch with us as we will be sharing more content in our future blog posts.
Thanks for reading.